BDF Parser (Python library)
#
IntroductionThis is a BDF (Glyph Bitmap Distribution; Wikipedia; Spec) format bitmap font file parser library in Python. It has Font
, Glyph
and Bitmap
classes providing more than 30 chainable API methods of parsing BDF fonts, getting their meta information, rendering text in any writing direction, adding special effects and manipulating bitmap images. It works seamlessly with PIL / Pillow and NumPy, and has detailed documentation / tutorials / API reference.
BDF Parser TypeScript (JavaScript) library (documentation; GitHub page; npm page; npm i bdfparser
) is a port of BDF Parser Python library (documentation; GitHub page; PyPI page; pip install bdfparser
). Both are written by Tom Chen and under the MIT License.
The BDF Parser TypeScript (JavaScript) library has a Live Demo & Editor you can try.
#
Installation#
Quick examplesPrint cropped and combined "a" and "c" with shadow effect:
Get an enlarged version (8 times both width and height) of this "ac":
And save it as an RGBA (background transparent) image "ac.png", using PIL (Pillow) library (pip install pillow
if needed):
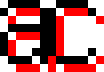
Print text "Hello!", from right to left, with glowing effect:
This time, try NumPy and Matplotlib! (pip install
if you don't have them)
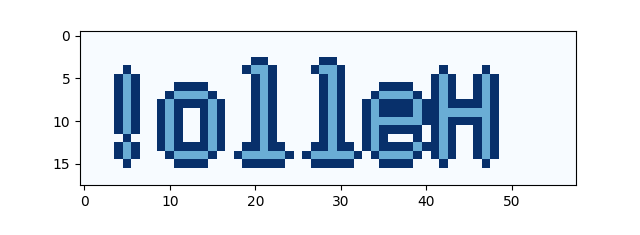
Save all glyphs in Unifont as a black-and-white-two-color-only "font_preview.png" (with default width 512px):
#
Copyright & linksWritten by Tom Chen, under the MIT License, a permissive open-source license.
This library officially supports Python version 3.5 or later.
The documentation belongs to font.tomchen.org, a website where I put font & typography related stuff. The documentation website's GitHub repo