Bitmap object
Bitmap()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
bin_bitmap_list | Required | list of strings | N/A | Binary bitmap data, which is a list of '0' /'1' /'2' strings |
#
Return valueBitmap object
#
DescriptionInitialize a Bitmap object. Load binary bitmap data (list of strings).
.bindata
#
#
Syntax#
Examples#
Typelist of strings
#
DescriptionThis attribute of a Bitmap object represents the raw binary data of the bitmap.
note
If you want to get the data, use API methods .todata() or .tobytes() instead.
.width()
#
#
Syntax#
Examples#
ParametersNo parameters
#
Return value(integer) Width of the bitmap
#
DescriptionGet the width of the bitmap.
.height()
#
#
Syntax#
Examples#
ParametersNo parameters
#
Return value(integer) Height of the bitmap
#
DescriptionGet the height of the bitmap.
.clone()
#
#
Syntax#
Examples#
ParametersNo parameters
#
Return value(Bitmap object) A deep copy of the original Bitmap object
#
DescriptionGet a deep copy / clone of the Bitmap object.
.crop()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
w | Required | integer | N/A | Width of the new bitmap |
h | Required | integer | N/A | Height of the new bitmap |
xoff | Optional | integer | 0 | Relative position in x (right) direction of the new starting (bottom-left) point from the old one |
yoff | Optional | integer | 0 | Relative position in y (top) direction of the new starting (bottom-left) point from the old one |
#
Return valueThe Bitmap object itself, which now has only the specified area as its .bindata
.
(Consider to use .clone()
first, if you want to maintain the original copy of the Bitmap object)
#
DescriptionCrop and/or extend the bitmap.
.overlay()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
bitmap | Required | Bitmap object | N/A | The incoming bitmap to overlay over the current one |
#
Return valueThe Bitmap object itself, which now has the combined bitmap as its .bindata
.
#
DescriptionOverlay another bitmap over the current one.
note
This method mutates the Bitmap object. Consider to use .clone() first, if you want to maintain the original copy of the Bitmap object.
Bitmap.concatall()
#
#
Syntax#
ExamplesClick to see the output of the above code
Click to see the output of the above code
Click to see the output of the above code
Click to see the output of the above code
Click to see the output of the above code
#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
bitmaplist | Required | list of Bitmap objects | N/A | list of bitmaps to concatenate |
direction | Optional | integer | 1 | 1 : right0 : down2 : left-1 : up |
align | Optional | integer | 1 | If horizontal (right (1 ) or left (2 )) direction:1 : bottom0 : topIf vertical (down ( 0 ) or up (-1 )) direction:1 : left0 : right |
offsetlist | Optional | list of integers | None | list of spacing offsets between every two glyphs: [offset_between_0_and_1, offset_between_1_and_2, ..., offset_between_2nd_last_and_last] . len(offsetlist) should be equal to len(bitmaplist)-1 . If None , then all offsets are 0 |
#
Return valueBitmap object
#
DescriptionConcatenate all Bitmap objects in a list.
This is a class / static method, meaning you need to call the method on the Bitmap
class: Bitmap.concatall()
+
concat#
#
Syntax#
Examples#
Description+
is a shortcut of Bitmap.concatall()
. Use +
to concatenate two Bitmap objects and get a new Bitmap objects.
It's equivalent to Bitmap.concatall([bitmap1, bitmap2])
, using default values of all the optional parameters of Bitmap.concatall()
. bitmap1
won't change
.concat()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
bitmap | Required | Bitmap object | N/A | Bitmap to concatenate |
offset | Required | integer | 0 | Spacing offset between the glyphs. See also offsetlist parameter in Bitmap.concatall() |
For the rest of the parameters (direction
, align
), see Bitmap.concatall()
's "Parameters" section
#
Return valueThe Bitmap object itself, which now has the combined bitmap as its .bindata
.
#
DescriptionConcatenate another Bitmap objects to the current one.
It is similar to bitmap1 + bitmap2
and Bitmap.concatall([bitmap1, bitmap2])
, but update the current Bitmap object and return it, instead of creating a new one like the other two methods.
note
This method mutates the Bitmap object. If you want to maintain the original copy of the Bitmap object, consider to use Bitmap.concatall(), or + sign, or use .clone() first.
.enlarge()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
x | Optional | integer | 1 | Multiplier in x (right) direction |
y | Optional | integer | 1 | Multiplier in y (top) direction |
#
Return valueThe Bitmap object itself, which now has the enlarged bitmap as its .bindata
.
#
DescriptionEnlarge a Bitmap object, by multiplying every pixel in x (right) direction and in y (top) direction.
*
enlarge#
#
Syntax#
Examples#
Description*
is a shortcut of .enlarge()
.
bitmap_object * i
is similar to bitmap_object.enlarge(i, i)
, but returns a new Bitmap object instead of updating the current one.
bitmap_object * (x, y)
is similar to bitmap_object.enlarge(x, y)
, but returns a new Bitmap object instead of updating the current one.
.replace()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
substr | Required | strings | N/A | Substring to be replaced |
newsubstr | Required | strings | N/A | New substring as the replacement |
#
Return valueThe Bitmap object itself, which now has the altered bitmap as its .bindata
.
#
DescriptionReplace a string by another in the bitmap. Because the bitmap's data is stored as a list of '0'
/'1'
/'2'
strings (e.g. ['0211','1010','0200']
), you can replace a substring (e.g. '2'
by '1'
) in it.
.shadow()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
xoff | Optional | integer | 1 | Shadow's offset in x (right) direction |
yoff | Optional | integer | -1 | Shadow's offset in y (top) direction |
#
Return valueThe Bitmap object itself, which now has a bitmap of the original shape with its shadow as the Bitmap object's .bindata
.
#
DescriptionAdd shadow to the shape in the bitmap.
The shadow will be filled by '2'
s.
Default parameters (xoff=1, yoff=-1
) mean add a shadow which is 1 pixel right and 1 pixel bottom to the original shape.
.glow()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
mode | Optional | integer | 0 | Mode. Corner pixels will not be filled in default mode 0 but will in mode 1 |
#
Return valueThe Bitmap object itself, which now has a bitmap of the original shape with glow effect as the Bitmap object's .bindata
.
#
DescriptionAdd glow effect to the shape in the bitmap.
The glowing area is one pixel up, right, bottom and left to the original pixels (corners will not be filled in default mode 0 but will in mode 1), and will be filled by '2'
s.
.bytepad()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
bits | Optional | integer | 8 | Each line should be padded to multiple of how many bits/pixels |
#
Return valueThe Bitmap object itself, which now has the altered bitmap as its .bindata
.
#
DescriptionPad each line (row) to multiple of 8 (or other numbers) bits/pixels, with '0'
s.
Do this before using the bitmap for a glyph in a BDF font: per BDF spec, if the bit/pixel count in a line is not multiple of 8, the line needs to be padded on the right with 0s to the nearest byte (that is, multiple of 8).
Parameter bits
is 8
by default because 1 byte = 8 bits, you can change it if you want to use other unconventional, off-spec values, such as 4 (half a byte).
.todata()
#
#
Syntax#
Examples#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
datatype | Optional | See below | 1 | Output data type. See below |
datatype
: output data type:
0
: binary-encoded multi-line string. e.g.'00010\n11010\n00201'
1
: list of binary-encoded strings. e.g.['00010','11010','00201']
2
: list of lists of integers0
or1
(or2
sometimes). e.g.[[0,0,0,1,0],[1,1,0,1,0],[0,0,2,0,1]]
3
: list of integers0
or1
(or2
sometimes) (to be used with.width()
and.height()
). e.g.[0,0,0,1,0,1,1,0,1,0,0,0,2,0,1]
4
: list of lowercase hexadecimal-encoded strings (without'0x'
, padded with leading'0'
s according to width). e.g.['02','1a']
5
: list of integers. e.g.[2,26]
note
You can't have '2'
s when using datatype
4
or 5
.
note
If you use NumPy, set datatype
to 2
. Below is a simple example, see "Quick examples" for another example with NumPy and Matplotlib.
#
Return valueBitmap data in the specified type (list or string) and format
#
DescriptionGet the bitmap's data in the specified type and format.
.tobytes()
#
#
Syntax#
Examples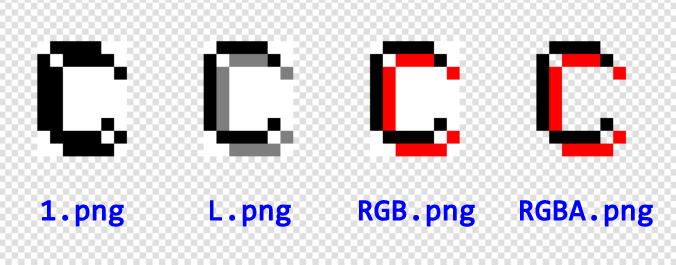
#
ParametersName | R/O | Type | Default Value | Description |
---|---|---|---|---|
mode | Optional | string | 'RGB' | PIL image mode. See below |
bytesdict | Optional | dictionary (integer as keys, bytes as values) or None | Varies with mode . See below | dictionary mapping 0 /1 /2 in the bitmap data to bytes |
mode
: PIL image mode, it can be:
'1'
: 1-bit pixels, black and white (two colors only), stored with one pixel per byte'L'
: 8-bit pixels, black and white (grayscale)'RGB'
: 3x8-bit pixels, RGB model, true color'RGBA'
: 4x8-bit pixels, RGBA model, true color with transparency mask
bytesdict
's default value varies with mode
:
mode='1'
:{ 0: 1, 1: 0, 2: 0 }
, this one is special, the values are not bytes, although the final output is. Value1
is white and0
is blackmode='L'
:{ 0: b'\xff', 1: b'\x00', 2: b'\x7f' }
. Valueb'\xff'
is white,b'\x00'
is black,b'\x7f'
is middle gray. You can select gray of other shadesmode='RGB'
:{ 0: b'\xff\xff\xff', 1: b'\x00\x00\x00', 2: b'\xff\x00\x00' }
. Valueb'\xff\xff\xff'
is white,b'\x00\x00\x00'
is black,b'\xff\x00\x00'
is red. The 3 bytes represent red, green, blue channels in the RGB modelmode='RGBA'
:{ 0: b'\xff\xff\xff\x00', 1: b'\x00\x00\x00\xff', 2: b'\xff\x00\x00\xff' }
. Same as'RGB'
, supplemented with a fourth byte representing alpha channel (opacity)
(see also the "Modes" section in Pillow's docs, but only the above modes are supported here)
note
If the bitmap is large (for example, it is what you got from Font object's .drawall(), then it would be better to use '1'
mode. Other modes, although have no issue with bdfparser's .tobytes()
method, they could be very slow when you load the data in bytes with Pillow library's Image.frombytes()
. Because default mode
is 'RGB'
, remember to specify '1'
in this case.
#
Return value(bytes) Bitmap data as bytes of the specified format
#
DescriptionSometimes you want to output bitmap data and load it with PIL / Pillow (Wikipedia; official site; docs; GitHub; PyPI), a Python imaging library.
This method helps you to get the bitmap's data as bytes to be used with Pillow's Image.frombytes(mode, size, data)
.
str()
and print()
#
#
Syntax#
Examples#
Description(string) str()
gets a human-readable (multi-line) string representation of the Bitmap object.
print()
prints a human-readable (multi-line) string representation of the Bitmap object.
The following digits in the bitmap's binary-encoded string list data are replaced in str()
and print()
's output:
'0'
s are replaced by#
'1'
s are replaced by.
'2'
s are replaced by&
repr()
#
#
Syntax#
Examples#
Description(string) It gets a programmer-readable (multi-line) string representation of the Bitmap object.